Stages of the Activity lifecycle | Android Developers
3. Explore the lifecycle methods and add basic logging
Every activity has what is known as a lifecycle. This term is an allusion to plant and animal lifecycles, like the lifecycle of a butterfly — the different states of the butterfly show its growth from egg to caterpillar to pupa to butterfly to death .
Similarly, the activity lifecycle consists of the different states that an activity can go through, from when the activity first initializes to its destruction, at which time the operating system (OS) reclaims its memory. Typically, the entry point of a program is the main()
method. Android activities, however, begin with the onCreate()
method; this method would be the equivalent of the egg stage in the above example. You have used activities already, many times throughout this course, and you might recognize the onCreate()
method. As the user starts your app, navigates between activities, navigates inside and outside of your app, the activity changes state.
The following diagram shows all the activity lifecycle states. As their names indicate, these states represent the status of the activity. Notice that, unlike the butterfly lifecycle, an activity can go back and forth between states throughout the lifecycle, instead of only moving in a single direction.
Bạn đang đọc: Stages of the Activity lifecycle | Android Developers
Note: An Android app can have multiple activities. However, it is recommended to have a single activity, and so far that is what you have been implementing in this course.
![]()
Often, you want to change some behavior, or run some code, when the activity lifecycle state changes. Therefore, the
Activity
class itself, and any subclasses ofActivity
such asComponentActivity
, implement a set of lifecycle callback methods. Android invokes these callbacks when the activity moves from one state to another, and you can override those methods in your own activities to perform tasks in response to those lifecycle state changes. The following diagram shows the lifecycle states along with the available overridable callbacks.
Note: The asterisk on the
onRestart()
method indicates that this method is not called every time the state transitions between Created and Started. It is only called ifonStop()
was called and the activity is subsequently restarted.It’s important to know when Android invokes the overridable callbacks and what to do in each callback method, but both of these diagrams are complex and can be confusing. In this codelab, instead of just reading what each state and callback means, you’re going to do some detective work and build your understanding of the Android activity lifecycle .
Step 1: Examine the
onCreate()
method and add loggingTo figure out what’s going on with the Android lifecycle, it’s helpful to know when the various lifecycle methods are called. This information helps you identify where things are going wrong in the Dessert Clicker app .
A simple way to determine this information is to use the Android logging functionality. Logging enables you to write short messages to a console while the app runs and use it to see when different callbacks are triggered .
- Run the Dessert Clicker app and tap several times on the picture of the dessert. Note how the value for Desserts sold and the total dollar amount changes.
- Open
MainActivity.kt
and examine theonCreate()
method for this activity:override fun onCreate(savedInstanceState: Bundle?) { // ... }
In the activity lifecycle diagram, you may recognize the
onCreate()
method, because you’ve used this callback before. It’s the one method that every activity must implement. TheonCreate()
method is where you should do any one-time initializations for your activity. For example, inonCreate()
, you callsetContent()
, which specifies the activity’s UI layout.
The
onCreate()
lifecycle method is called once, just after the activity initializes—when the OS creates the newActivity
object in memory. AfteronCreate()
executes, the activity is considered created.Note: When you override the
onCreate()
method, you must call the superclass implementation to complete the creation of the Activity, so within it, you must immediately callsuper.onCreate()
. The same is true for other lifecycle callback methods.
- Add the following constant at the top level of the
MainActivity.kt
, above the class declarationclass MainActivity
.A good convention is to declare a
TAG
constant in your file as its value will not change.To mark it as a compile-time constant, use
const
when declaring the variable. A compile-time constant is a value that is known during compilation.Xem thêm: Top 10 phần mềm quay video trên laptop
private const val TAG = "MainActivity"
- In the
onCreate()
method, just after the call tosuper.onCreate()
, add the following line:Log.d(TAG, "onCreate Called")
- Import the
Log
class, if necessary (pressAlt+Enter
, orOption+Enter
on a Mac, and select Import.) If you enabled auto imports, this should happen automatically.import android.util.Log
The
Log
class writes messages to the Logcat. The Logcat is the console for logging messages. Messages from Android about your app appear here, including the messages you explicitly send to the log with theLog.d()
method or otherLog
class methods.There are three important aspects of the
Log
instruction:
- The priority of the log message, that is, how important the message is. In this case, the
Log.v()
logs verbose messages.Log.d()
method writes a debug message. Other methods in theLog
class includeLog.i()
for informational messages,Log.w()
for warnings, andLog.e()
for error messages.- The log
tag
(the first parameter), in this case"MainActivity"
. The tag is a string that lets you more easily find your log messages in the Logcat. The tag is typically the name of the class.- The actual log message, called
msg
(the second parameter), is a short string, which in this case is"onCreate Called"
.
- Compile and run the Dessert Clicker app. You don’t see any behavior differences in the app when you tap the dessert. In Android Studio, at the bottom of the screen, click the Logcat tab.
- In the Logcat window, type
tag:MainActivity
into the search field.
The Logcat can contain many messages, most of which aren’t useful to you. You can filter the Logcat entries in many ways, but searching is the easiest. Because you used
MainActivity
as the log tag in your code, you can use that tag to filter the log. Your log message includes the date and time, your log tag, the name of the package (com.example.dessertclicker
), and the actual message. Because this message appears in the log, you know thatonCreate()
was executed.Step 2: Implement the
onStart()
methodThe
onStart()
lifecycle method is called just afteronCreate()
. AfteronStart()
runs, your activity is visible on the screen. UnlikeonCreate()
, which is called only once to initialize your activity,onStart()
can be called by the system many times in the lifecycle of your activity.
Note that
onStart()
is paired with a correspondingonStop()
lifecycle method. If the user starts your app and then returns to the device’s home screen, the activity is stopped and is no longer visible on screen.
- In Android Studio, with
MainActivity.kt
open and the cursor within theMainActivity
class, select Code > Override Methods… or pressControl+O
. A dialog appears with a long list of all the methods you can override in this class.
- Start entering
onStart
to search for the correct method. To scroll to the next matching item, use the down arrow. ChooseonStart()
from the list and click OK to insert the boilerplate override code. The code looks like the following example:override fun onStart() { super.onStart() }
- Inside the
onStart()
method, add a log message:override fun onStart() { super.onStart() Log.d(TAG, "onStart Called") }
- Compile and run the Dessert Clicker app and open the Logcat pane.
- Type
tag:MainActivity
into the search field to filter the log. Notice that both theonCreate()
andonStart()
methods were called one after the other, and that your activity is visible on screen.- Press the Home button on the device and then use the Recents screen to return to the activity. Notice that the activity resumes where it left off, with all the same values, and that
onStart()
is logged a second time to Logcat. Notice also that theonCreate()
method is not called again.2023-04-11 11:23:38.116 3275-3275 MainActivity com.example.dessertclicker D onCreate Called 2023-04-11 11:23:38.161 3275-3275 MainActivity com.example.dessertclicker D onStart Called 2023-04-11 11:24:16.721 3275-3275 MainActivity com.example.dessertclicker D onStart CalledNote: As you experiment with your device and observe the lifecycle callbacks, you might notice unusual behavior when you rotate your device. You’ll learn about that behavior later in this codelab.
Step 3: Add more log statements
In this step, you implement logging for all the other lifecycle methods .
- Override the remainder of the lifecycle methods in your
MainActivity
and add log statements for each one, as shown in the following code:override fun onResume() { super.onResume() Log.d(TAG, "onResume Called") } override fun onRestart() { super.onRestart() Log.d(TAG, "onRestart Called") } override fun onPause() { super.onPause() Log.d(TAG, "onPause Called") } override fun onStop() { super.onStop() Log.d(TAG, "onStop Called") } override fun onDestroy() { super.onDestroy() Log.d(TAG, "onDestroy Called") }
- Compile and run Dessert Clicker again and examine Logcat.
Notice that this time, in addition to
onCreate()
andonStart()
, there’s a log message for theonResume()
lifecycle callback.2023-04-11 11:28:21.145 4244-4244 MainActivity com.example.dessertclicker D onCreate Called 2023-04-11 11:28:21.187 4244-4244 MainActivity com.example.dessertclicker D onStart Called 2023-04-11 11:28:21.189 4244-4244 MainActivity com.example.dessertclicker D onResume CalledWhen an activity starts from the beginning, you see all three of these lifecycle callbacks called in order :
onCreate()
when the system creates the app.onStart()
makes the app visible on the screen, but the user is not yet able to interact with it.onResume()
brings the app to the foreground, and the user is now able to interact with it.Despite the name, the
onResume()
method is called at startup, even if there is nothing to resume.
Source: https://thomaygiat.com
Category : Ứng Dụng
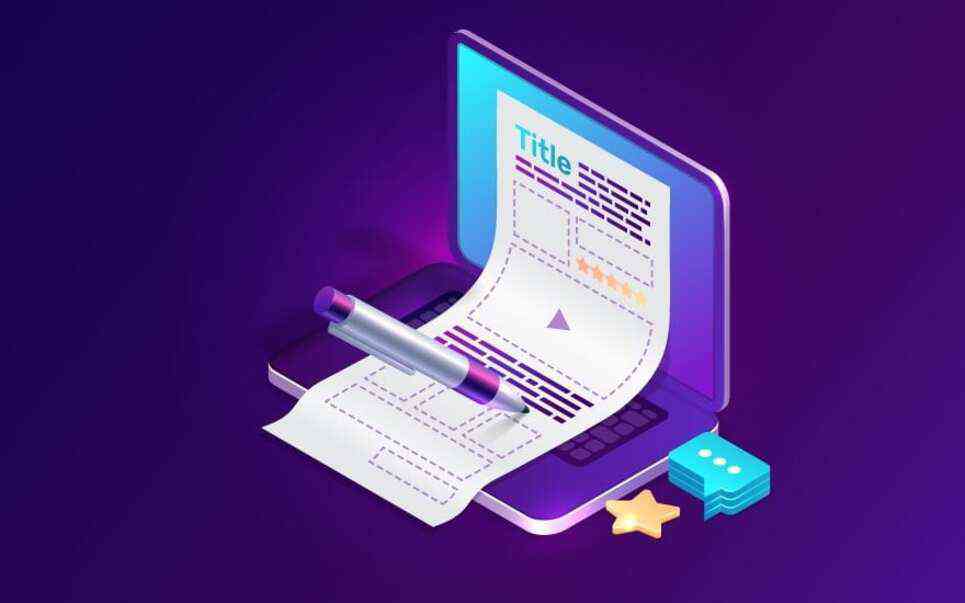
Nguyên nhân tủ lạnh Hitachi báo lỗi F0-17 và cách khắc phục
Mục ChínhNguyên nhân tủ lạnh Hitachi báo lỗi F0-17 và cách khắc phục1. Lỗi F017 trên tủ lạnh Hitachi là gì?2. Nguyên nhân gây ra…
Cách sửa máy điều hòa Sumikura báo lỗi chuẩn an toàn
Mục ChínhCách sửa máy điều hòa Sumikura báo lỗi chuẩn an toànNhận Biết Các Sự Cố và Lỗi Trên Điều Hòa SumikuraHướng Dẫn Kiểm Tra…
Hiểu cách sửa mã lỗi F0-16 Trên Tủ lạnh Hitachi Side By Side
Hiểu cách sửa mã lỗi F0-16 Trên Tủ lạnh Hitachi Side By Side https://appongtho.vn/tu-lanh-noi-dia-nhat-hitachi-bao-loi-f0-16 Tủ lạnh Hitachi là một thương hiệu uy tín được nhiều…
Cùng sửa điều hòa Gree báo lỗi chuẩn an toàn với App Ong Thợ
Mục ChínhCùng sửa điều hòa Gree báo lỗi chuẩn an toàn với App Ong ThợCó nên tự sửa mã lỗi điều hòa Gree?A: Khi Nên…
Tự sửa lỗi điều hòa Toshiba cùng ứng dụng Ong Thợ
Tự sửa lỗi điều hòa Toshiba cùng ứng dụng Ong Thợ https://appongtho.vn/ma-loi-dieu-hoa-toshiba Khi máy điều hòa Toshiba của bạn gặp sự cố, việc tự kiểm…
15+ app hack game đỉnh cao giúp bạn làm chủ mọi cuộc chơi
Cùng với sự tăng trưởng của ngành công nghiệp game, những app hack game không tính tiền cũng tăng trưởng theo để giúp người chơi…