Cách mã hóa – \”Băm\” mật khẩu trong Nodejs bằng Bcrypt > Ironhack Việt Nam
Băm là một tấm vé một chiều để mã hóa dữ liệu. Hashing triển khai quy đổi một chiều trên mật khẩu, biến mật khẩu thành một chuỗi khác, được gọi là mật khẩu băm .
Một số điều kiện để chúng ta có thể bắt đầu
Đây là bài viết dành cho những bạn đã có những khái niệm cơ bản về JavaScript, Nodejs và MongoDB :
Đảm bảo rằng bạn đã cài đặt phần mềm sau trên hệ thống của mình:
Bạn đang đọc: Ironhack Việt Nam”>Cách mã hóa – \”Băm\” mật khẩu trong Nodejs bằng Bcrypt > Ironhack Việt Nam
Không dài dòng thêm nữa, chúng ta cùng bắt đầu
Bước 1 : Tạo một dự án Nodejs mới và tiến hành cài đặt init
npm init -y
Bước 2 : Cài đặt Express.js và Mongoose
npm install --save mongoose express
Bước 3 : Setup Express.js và kết nối nó với MongoDB bằng mongoose.
- Tạo một tệp mới có tên là index.js tại thư mục gốc của dự án và thêm đoạn code dưới đây vào:
const { request, response } = require("express"); const express = require("express"); const mongoose = require("mongoose"); const app = express(); const port = 8000; const userRouter = require("./app/routers/userRouter") const path = require("path"); app.use(express.json()); app.use(express.urlencoded({ extended: true })); mongoose.connect("mongodb://localhost:27017/Test", (error) => { if (error) throw (error) console.log("Successfulity connect to MongoDB") }) app.listen(port, () => { console.log("App lisstening on port " + port) })
Như vậy, tất cả chúng ta đã tạo một dự án Bất Động Sản mới trong MongoDB tên là Test và một sever trên cổng 8000 .
Bước 4 Tiếp theo, tạo một thư mục app, trong đó chứa các components con là controller, model, router
Tại thư mục Model, tạo file userModel. js và thêm đoạn code sau :const mongoose = require("mongoose"); const Schema = mongoose.Schema; const userSchema = new Schema({ username: { type: String, required: true }, email: { type: String, required: true, unique: true, match: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i }, password: { type: String, required: true, }, createdAt: { type: Date, default: Date.now() }, updatedAt: { type: Date, default: Date.now() } }) module.exports = mongoose.model("User", userSchema)
Cài đặt bcrypt
npm i --save bcrypt
Tại thư mục Controller, tạo file userController. js và thêm đoạn code sau :
const bcrypt = require("bcrypt"); const userModel= require("../models/userModel"); const mongoose = require("mongoose"); const { request } = require("express"); const { response } = require("express"); const validatePasword = (password) => { return password.match( // Tối thiểu tám ký tự, ít nhất một chữ cái, một số và một ký tự đặc biệt /^(?=.*[A-Za-z])(?=.*\d)(?=.*[@$!%*#?&])[A-Za-z\d@$!%*#?&]{8,}$/ ); }; const createUser = async (request, response) => { let bodyRequest = request.body; if(!bodyRequest.username) { return response.status(400).json({ status: "Error 400: Bad Request", message: "username is required" }) } if(!bodyRequest.email) { return response.status(400).json({ status: "Error 400: Bad Request", message: "email is required" }) } if(!bodyRequest.password) { return response.status(400).json({ status: "Error 400: Bad Request", message: "password is required" }) } if (!validatePasword(bodyRequest.password)) { return response.status(400).json({ status: "Error 400: Bad Request", message: "password is not valid" }) } let user = new userModel(bodyRequest); const salt = await bcrypt.genSalt(10); user.password = await bcrypt.hash(user.password, salt); user.save((error, doc) => { if(error) { response.status(500).json({ status: "Error 500: Email đã được đăng ký", message: error.message }) } else { response.status(201).send({ status: "Success 201: Đăng ký thành công", doc: doc }) } }) } const logInUser = async (request, response) => { let bodyRequest = request.body; const user = await userModel.findOne({ email: bodyRequest.email }); if (user) { const validPassword = await bcrypt.compare(bodyRequest.password, user.password); if (validPassword) { response.status(200).json({ status: "ok" }); } else { response.status(400).json({ error: "Password không đúng!!!" }); } } else { response.status(401).json({ error: "Email không đúng!!!" }); } } module.exports = { createUser, logInUser }
Tại thư mục router, tạo file userRouter. js và thêm đoạn code sau :
const express = require("express") const { createUser, logInUser } = require("../controller/userController") const router = express.Router(); router.post("/register", createUser); router.post("/login", logInUser); // exports router module.exports = router;
Bước 5 : Chạy thử bằng Postman
Chạy dự án bằng lệnh node index.js.
Tạo người dùng mới với một email và mật khẩu bằng cách gửi yêu cầu Post tại
http://localhost:8000/register
. Lưu ý rằng bạn sẽ nhận được mật khẩu băm trong MogooDB.Thự hiện đăng nhập bằng cách gửi yêu cầu Post tại
http://localhost:8000/login
.Kết quả trong MogooDB sẽ như thế này:
Xem thêm: Lịch sử Internet – Wikipedia tiếng Việt
![]()
Chúc những bạn thành công xuất sắc .
Cựu học viên Ironhack Việt Nam – Hiện là full-stack Developer tại All Xone
Source: https://thomaygiat.com
Category : Kỹ Thuật Số
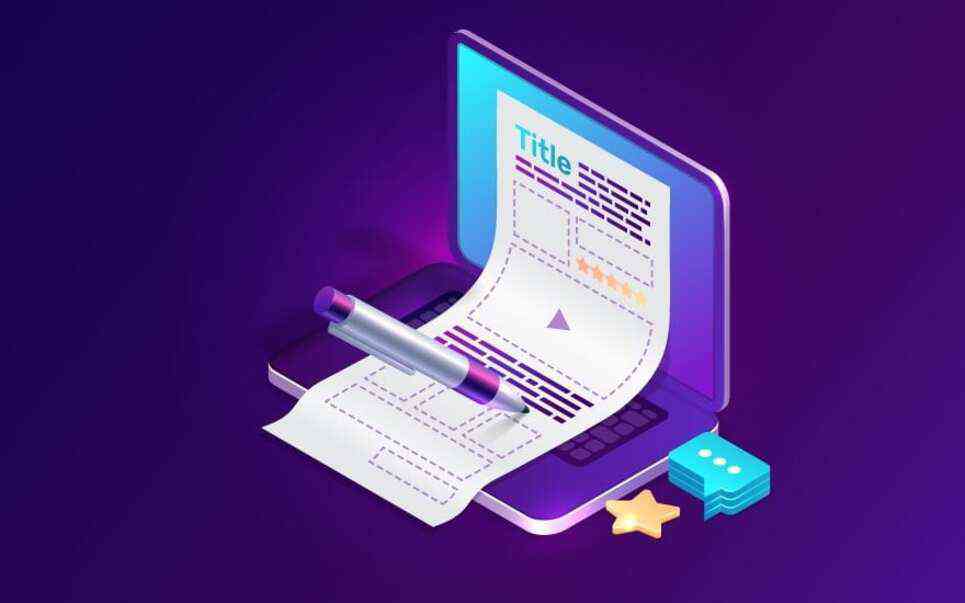
Chuyển vùng quốc tế MobiFone và 4 điều cần biết – MobifoneGo
Muốn chuyển vùng quốc tế đối với thuê bao MobiFone thì có những cách nào? Đừng lo lắng, bài viết này của MobiFoneGo sẽ giúp…
Cách copy dữ liệu từ ổ cứng này sang ổ cứng khác
Bạn đang vướng mắc không biết làm thế nào để hoàn toàn có thể copy dữ liệu từ ổ cứng này sang ổ cứng khác…
Hướng dẫn xử lý dữ liệu từ máy chấm công bằng Excel
Hướng dẫn xử lý dữ liệu từ máy chấm công bằng Excel Xử lý dữ liệu từ máy chấm công là việc làm vô cùng…
Cách nhanh nhất để chuyển đổi từ Android sang iPhone 11 | https://thomaygiat.com
Bạn đã mua cho mình một chiếc iPhone 11 mới lạ vừa ra mắt, hoặc có thể bạn đã vung tiền và có một chiếc…
Giải pháp bảo mật thông tin trong các hệ cơ sở dữ liệu phổ biến hiện nay
Hiện nay, với sự phát triển mạnh mẽ của công nghệ 4.0 trong đó có internet và các thiết bị công nghệ số. Với các…
4 điều bạn cần lưu ý khi sao lưu dữ liệu trên máy tính
08/10/2020những chú ý khi tiến hành sao lưu dữ liệu trên máy tính trong bài viết dưới đây của máy tính An Phát để bạn…